정렬과 배치
은근히 헷갈리지만 간단한 flexDirection
, justifyContent
와 alignItems
를 활용한 정렬과 배치에 대해 알아보자.
Flex Direction
Flex Direction은 View의 자식요소들을 가로 혹은 세로, 어떤 방향으로 배치할지를 정의해 준다.
flexDirection: 'row'
는 가로 배치를, flexDirection: 'column'
은 세로 배치를 뜻하는데, 기본값이 'column'
으로 되어있기 때문에 따로 명시해 주지 않으면 View의 자식요소들이 세로로 배치되게 된다.
import React from 'react';
import { View, StyleSheet } from 'react-native';
export default class StatScreen extends React.Component {
render() {
return (
<View style={styles.container}>
<View style={styles.red} />
<View style={styles.yellow} />
<View style={styles.green} />
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row' // 혹은 'column'
},
red: { flex: 1, backgroundColor: '#c94045' },
yellow: { flex: 1, backgroundColor: '#e2a74b' },
green: { flex: 1, backgroundColor: '#7abb7d' }
});
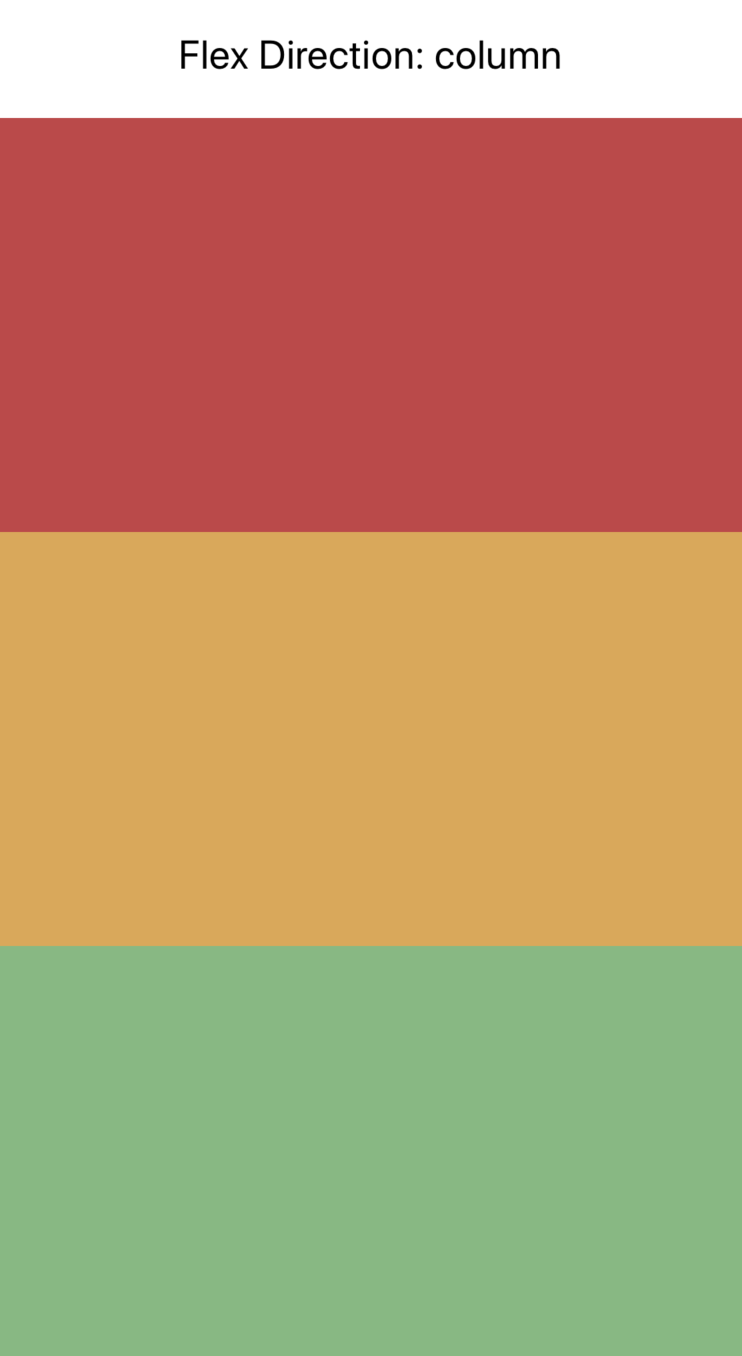
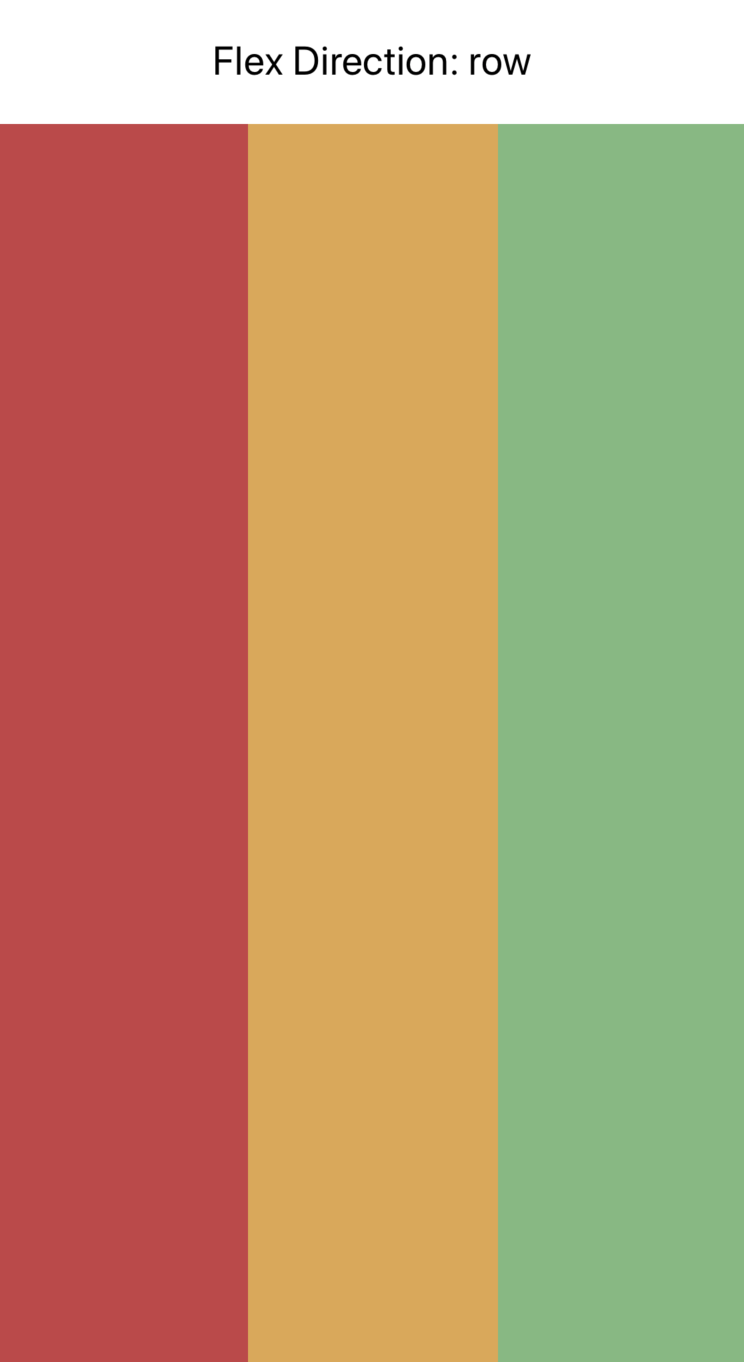
Justify Content
Justify Content는 Flex Direction과 동일한 방향으로의 정렬방법이다. flex-start
, flex-end
, center
, space-between
그리고 space-around
이 있으며, flex-start
가 디폴트 값이다. space-between
는 자식요소들을 동일한 간격으로 양끝정렬 하는 것으로 유용하게 쓰일 수 있다.
아래 예시는 flexDirection: 'row'
일 때, justifyContent: 'space-between'
속성을 주면 요소들이 가로 방향으로 동일한 간격으로 양끝정렬되는 것을 확인할 수 있다.
import React from 'react';
import { View, StyleSheet } from 'react-native';
export default class StatScreen extends React.Component {
render() {
return (
<View style={styles.container}>
<View style={styles.red} />
<View style={styles.yellow} />
<View style={styles.green} />
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row',
justifyContent: 'space-between'
},
red: { width: 50, height: 50, backgroundColor: '#c94045' },
yellow: { width: 50, height: 50, backgroundColor: '#e2a74b' },
green: { width: 50, height: 50, backgroundColor: '#7abb7d' }
});
Align Items
Justify Content와 반대로, Align Item는 Flex Direction 수직 방향으로의 정렬 속성이다.
위의 예시에 alignItems: 'center'
를 추가하면, 아래의 결과를 얻을 수 있다. stretch
, flex-start
, flex-end
, center
, 그리고 baseline
이 있으며, stretch
가 디폴트 값이다.
참고자료
👩🏻💻 배우는 것을 즐기는 프론트엔드 개발자 입니다
부족한 블로그에 방문해 주셔서 감사합니다 🙇🏻♀️
in the process of becoming the best version of myself